表操作
创建表 CREATE
创建表之前需要先切换到对应的数据库,比如 school:
USE school;
然后再编写创建表的 SQL 语句,语法如下:
CREATE TABLE [IF NOT EXISTS] table_name (
column1 data_type column_constraint,
column2 data_type,
...,
table_constraint,
...,
) ENGINE=storage_engine;
常见的约束包括主键、外键、唯一、非空、检查约束、默认值。
例如创建一个 student 数据表:
CREATE TABLE student (
`id` INT NOT NULL PRIMARY KEY,
`name` VARCHAR(20) NOT NULL,
`gender` BOOLEAN NOT NULL DEFAULT 1,
`birth` DATE,
);
- 数据库里面可以放表、函数、视图和过程,所以有些开发者喜欢将表名以
t
,tbl
,tb_
开头。 VARCHAR(20)
表示最大长度 20 个字符,一个汉字一个字符gender
最后不要用BOOLEAN
,因为 0/1 指代不明(不是 True/False),可以用但不是最好的选择,boolean 最终还是一位的整数。
一般建表的时候都尽量让每一个字段都不要为空,因为空值将来处理的时候会比较麻烦,会引发一些莫名其妙的问题。
能够唯一确定一条学生的列就把它称为主键,在这里就是学生的 id,如果想要创建一个自定义名称的主键约束,也可以使用表级约束,例如:
CREATE TABLE student (
`id` INT NOT NULL,
`name` VARCHAR(20) NOT NULL,
`gender` BOOLEAN NOT NULL DEFAULT 1,
`birth` DATE,
CONSTRAINT pk_id PRIMARY KEY (id)
);
删除表 DROP
DROP TABLE IF EXISTS student;
If you would like to drop the table, you can just right click the table and select Delete...
:
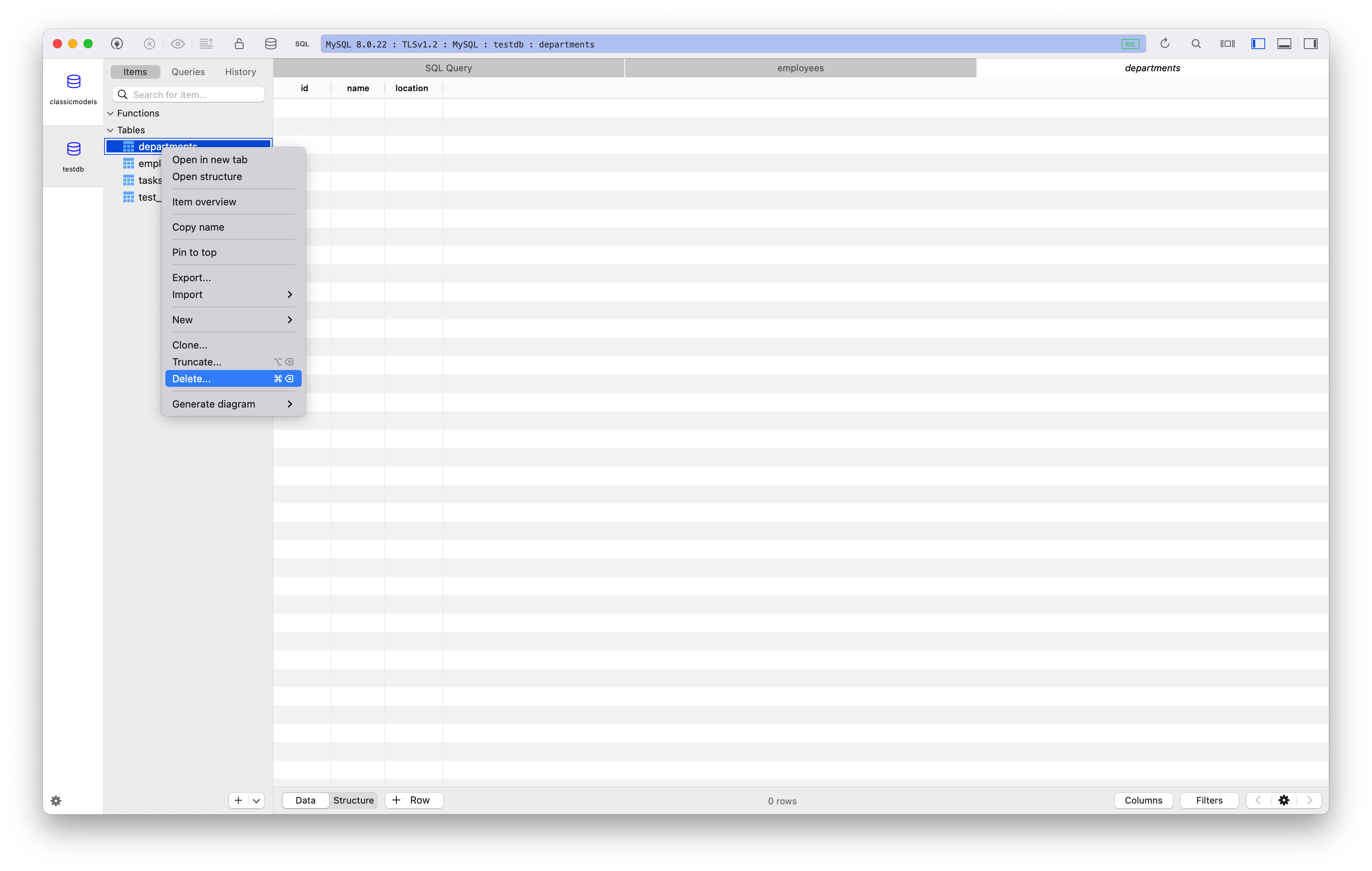
After that, there will be shown a dialog with some options as below. Since we are using MySQL, only ignore foreign key checks
can work fine.
You'd better not use shortcut which will drop table directly without showing dialog.
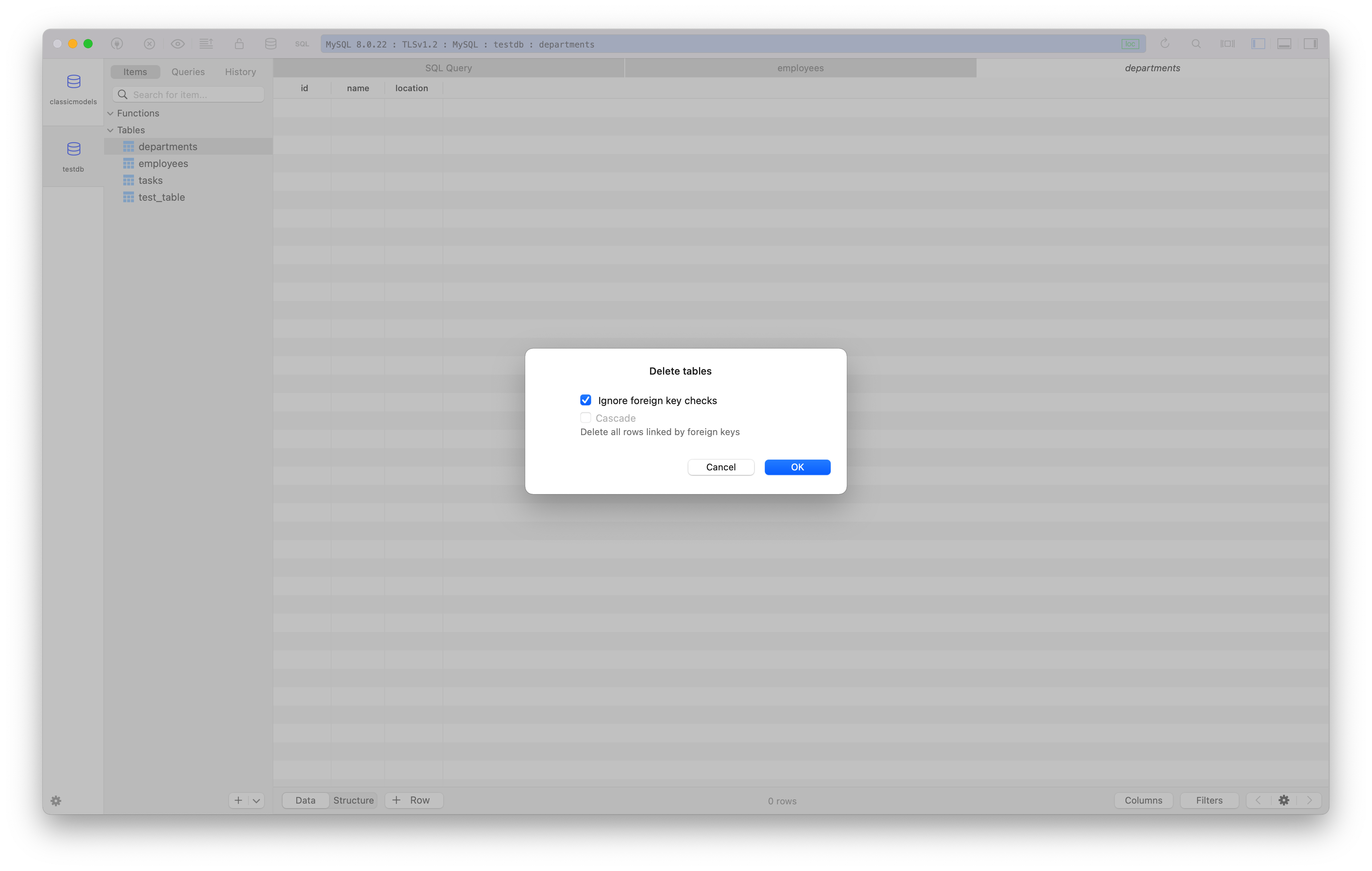
At last, you need to commit this change as well:
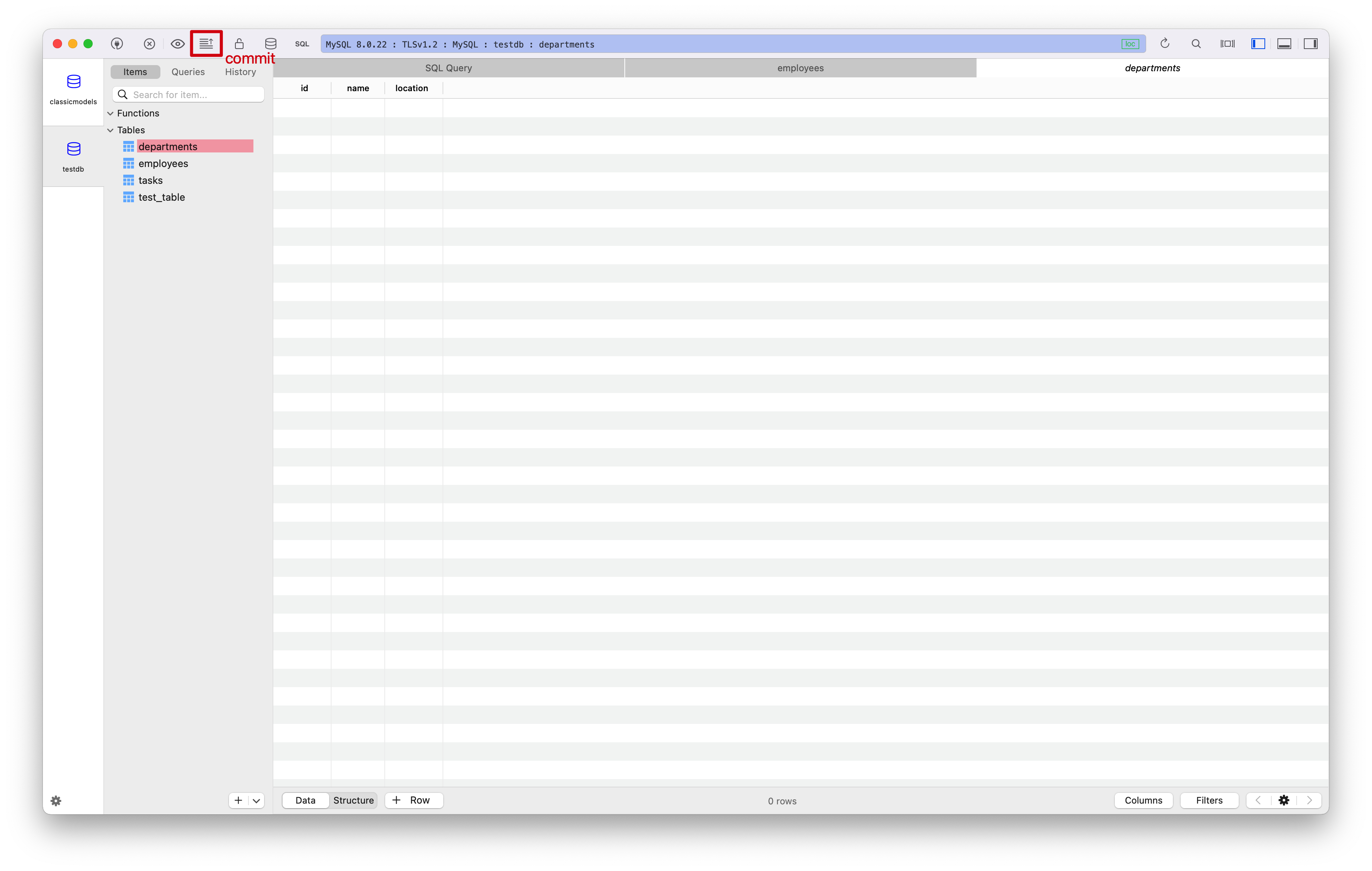
修改表 ALTER
如果原来表中有数据,不能破坏以前数据的完整性。
添加列
比如添加一个家庭住址:
ALTER TABLE student ADD COLUMN address VARCHAR(200) NOT NULL;
如果表中有数据,上面这样写是错误的,因为有非空约束,和以前的数据就产生了矛盾,可以加一个默认值:
ALTER TABLE student ADD COLUMN address VARCHAR(200) NOT NULL DEFAULT '';
删除列
ALTER TABLE student DROP COLUMN address;
修改列的数据类型
ALTER TABLE student MODIFY COLUMN gender ENUM ('男', '女') NOT NULL;
ENUM 是 mysql 的方言,其他许多数据库不支持,我们先改成 CHAR(1)
:
ALTER TABLE student MODIFY COLUMN gender CHAR(1) NOT NULL DEFAULT '男';
添加检查约束
ALTER TABLE student ADD CONSTRAINT ck_student_gender CHECK (gender = '男' OR gender = '女');
CHECK
其他许多数据也支持,MySQL ≥ 8.0.16 才支持。
修改列的名称
后面除了老新名字还必须要有类型,否则会报语法错误:
ALTER TABLE student CHANGE COLUMN gender stu_gender CHAR(1) NOT NULL DEFAULT '男';
如果还报以下错误是因为该列有约束:
Query 1 ERROR: Check constraint 'ck_student_gender' uses column 'gender', hence column cannot be dropped or renamed.
删除检查约束
ALTER TABLE student DROP CONSTRAINT ck_student_gender;
再修改名字就可以了。
查看表 SHOW
SHOW TABLES;
After creating tables by SQL statement, we can check the definition of table. In MySQL, using DESCRIBE
and SHOW CREATE TABLE
statements to show the table structure, see as follows:
Show the basic structure
DESCRIBE
or DESC
statement can show the field info including column name, type, is primary and default value. Syntax as following shows:
DESCRIBE <table_name>;
or
DESC <table_name>;
For example, let’s look at the products
table structure in the sample database.
DESC products;
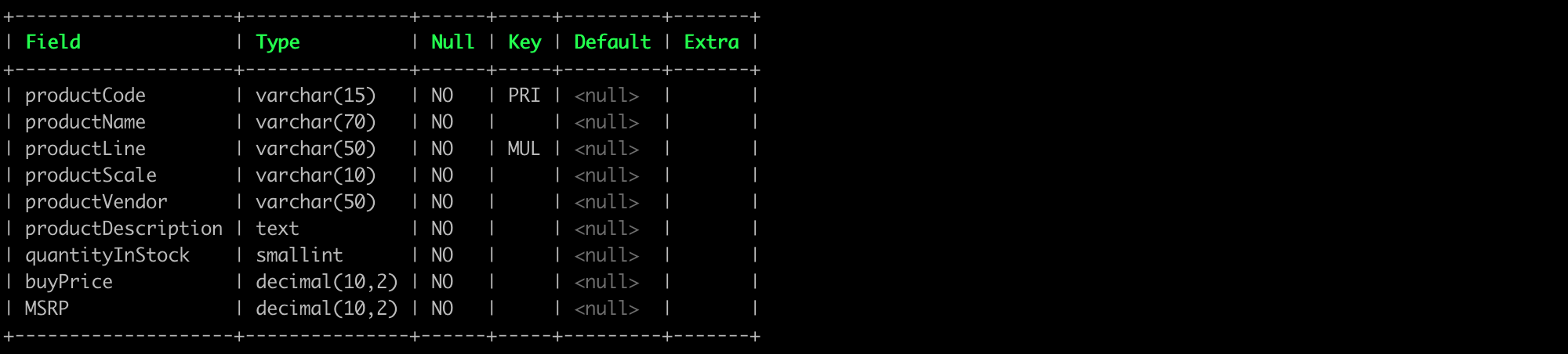
Show the detailed structure
This statement can show the CREATE TABLE
SQL statements when creating table, syntax as follows:
SHOW CREATE TABLE <table_name>\G;
When using SHOW CREATE TABLE
, it can not only show the detailed statements, but also shows the store engines and character encoding.
Adding \G
to make the result more visible:
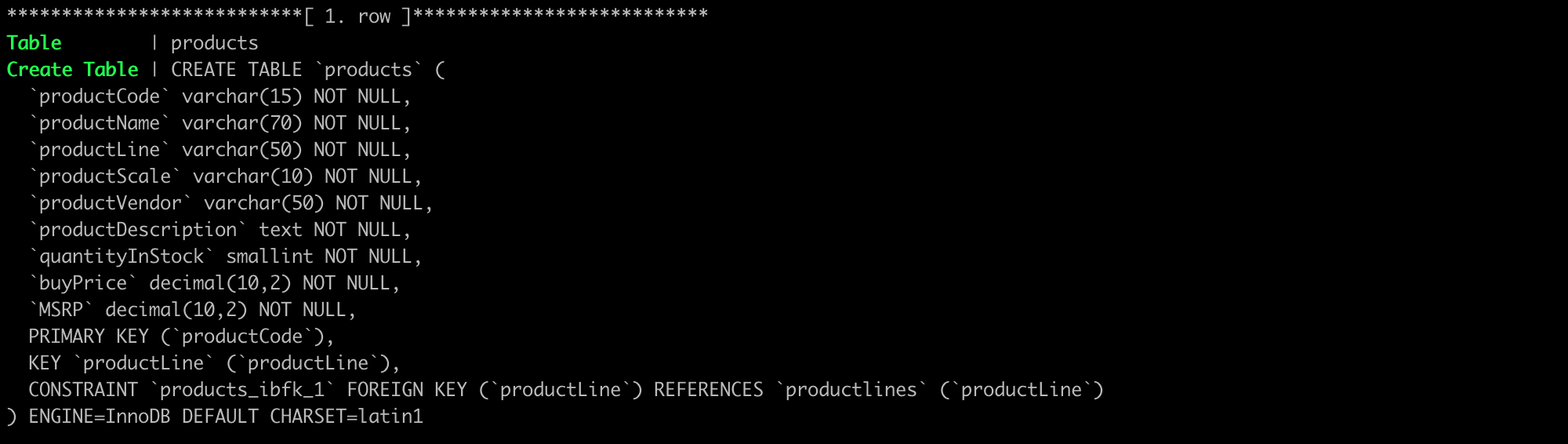
It's very simple to show table structure in TablePlus, follow the steps as below shows:
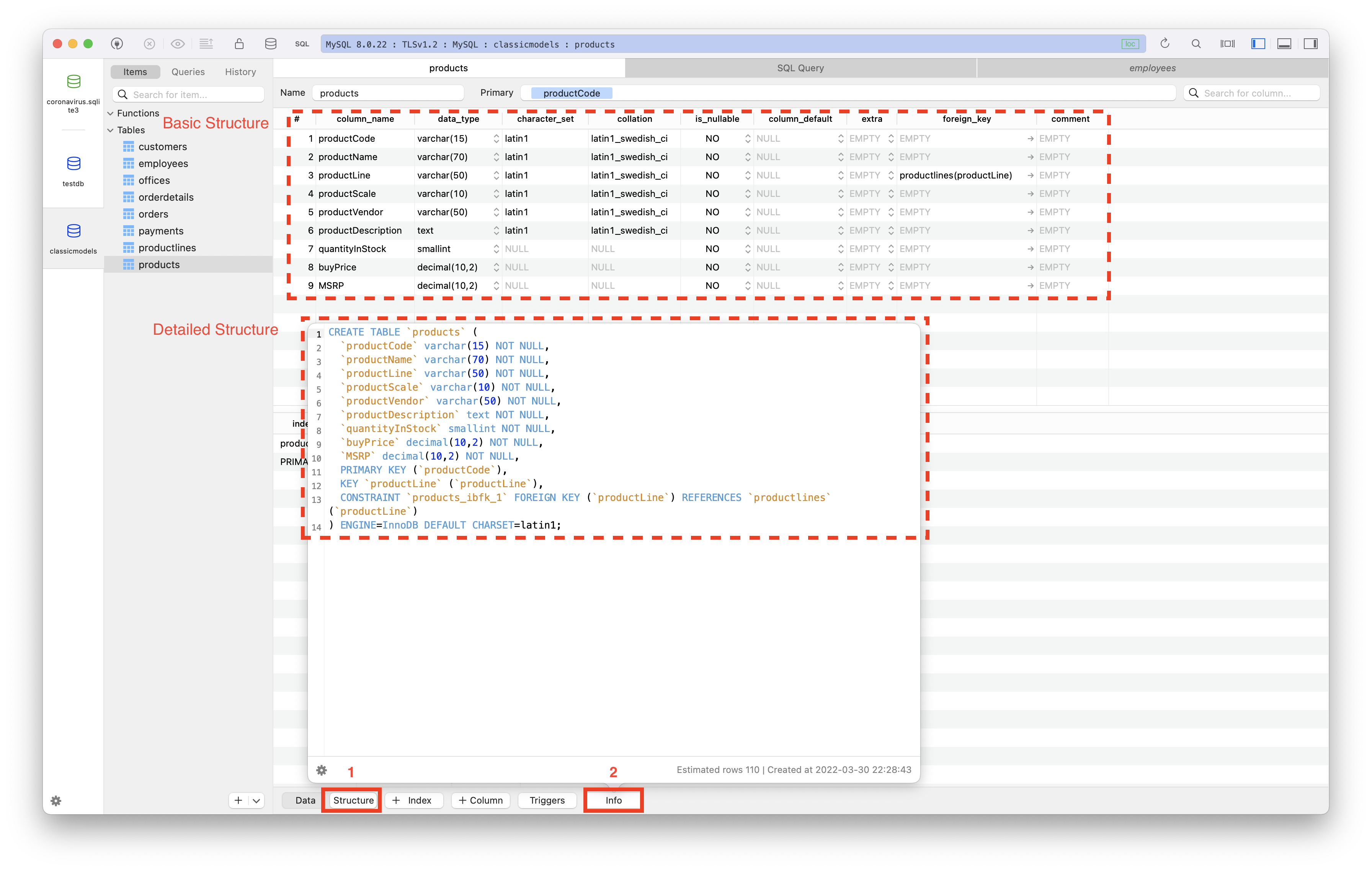
You can run SQL statements in TablePlus as well:
