Get Started
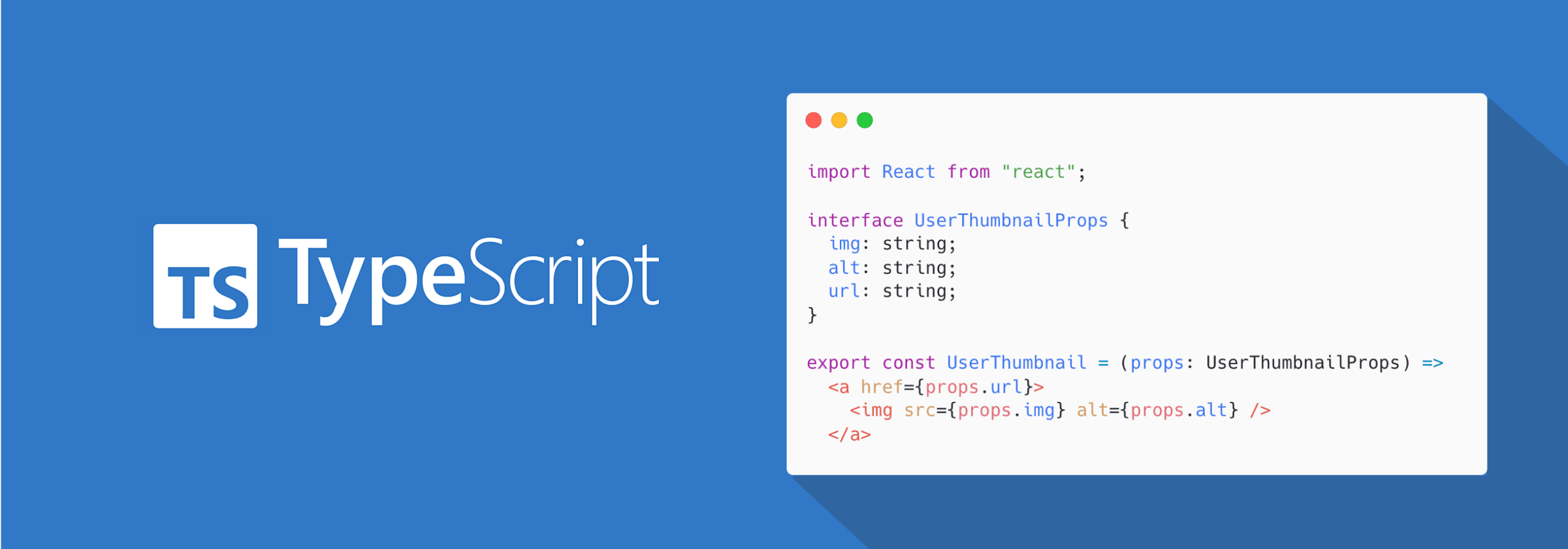
Install TypeScript
Let’s get started by building a simple web application with TypeScript.
Installing TypeScript
- npm
- Yarn
- pnpm
npm install -g typescript
yarn global add typescript
pnpm add -g typescript
Then you can check installation via:
tsc -v
Init the project
tsc --init
It will generate tsconfing.json
in the root of current directory as following shows:
{
"compilerOptions": {
"target": "es5" /* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017', 'ES2018', 'ES2019', 'ES2020', or 'ESNEXT'. */,
"module": "commonjs" /* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', 'es2020', or 'ESNext'. */,
"strict": true /* Enable all strict type-checking options. */,
"esModuleInterop": true /* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */,
"forceConsistentCasingInFileNames": true /* Disallow inconsistently-cased references to the same file. */
}
}
You can find more details in this chapter.
Above config is automatically generated by tsc --init
while it's not the default config of typescript.
Building your first TypeScript file
In your editor, type the following JavaScript code in greeter.ts:
function greeter(person) {
return 'Hello, ' + person;
}
let user = 'Jane User';
document.body.textContent = greeter(user);
Compiling your code
We used a .ts
extension, but this code is just JavaScript. You could have copy/pasted this straight out of an existing JavaScript app.
At the command line, run the TypeScript compiler:
tsc greeter.ts
The result will be a file greeter.js
which contains the same JavaScript that you fed in. We’re up and running using TypeScript in our JavaScript app!
Now we can start taking advantage of some of the new tools TypeScript offers. Add a : string
type annotation to the ‘person’ function argument as shown here:
function greeter(person: string) {
return 'Hello, ' + person;
}
let user = 'Jane User';
document.body.textContent = greeter(user);
Switch version of TypeScript
VS Code
If your workspace has a specific TypeScript version, you can switch between the workspace version of TypeScript and the version that VS Code uses by default.
First, opening a TypeScript or JavaScript file and clicking on the TypeScript version number in the status bar:

A message box will appear asking you which version of TypeScript VS Code should use:
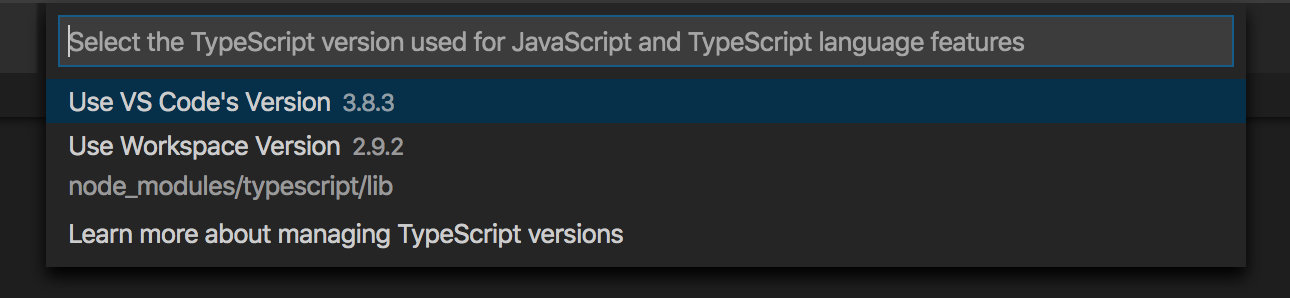
VS Code will automatically detect workspace versions of TypeScript that are installed under node_modules
in the root of your workspace.
To get a specific TypeScript version, specify @version
during npm install. For example, for TypeScript 2.9.2, you would use npm install --save-dev typescript@2.9.2
.
If TypeScript version < 2.x, it may occur some errors when switching version.
JetBrains
Click the status bar called TypeScript
:
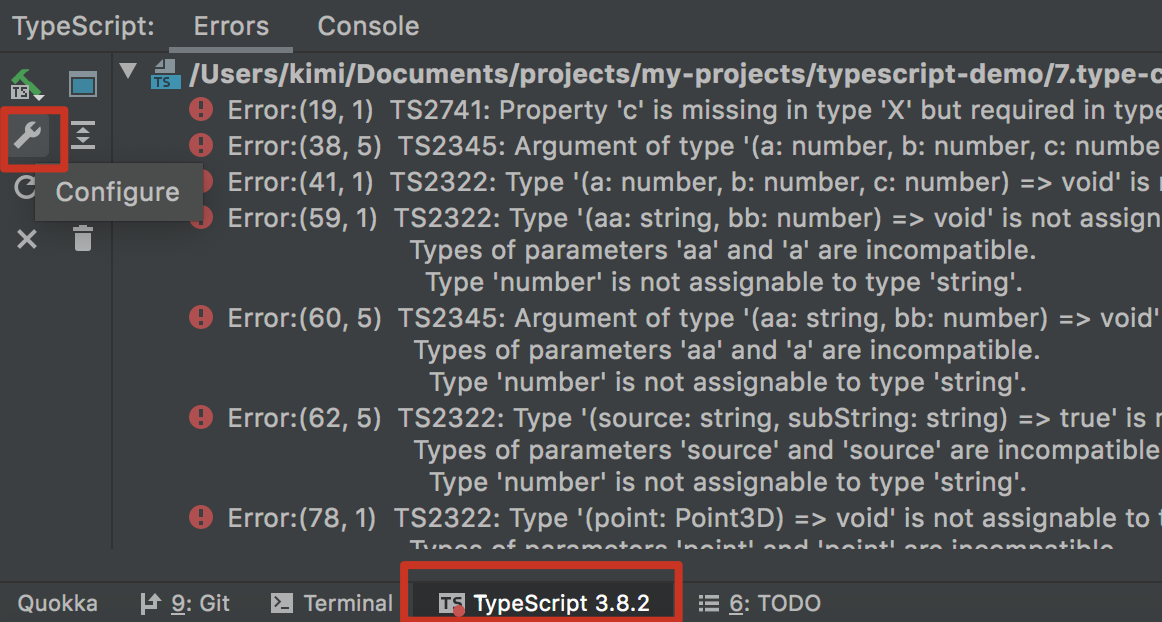
Select the path of the TypeScript:
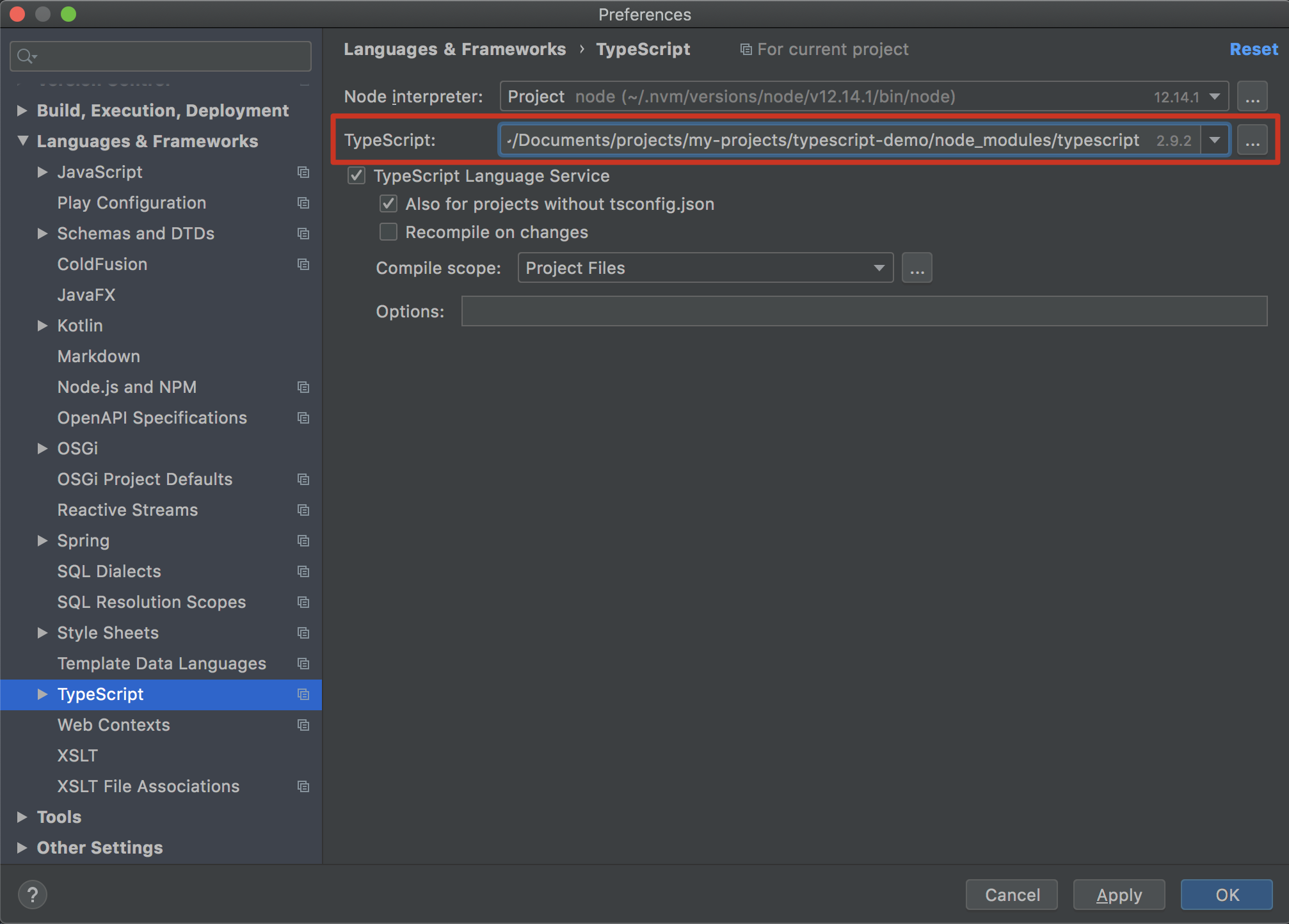
Demo
All demos are in this repo: https://github.com/tmf-map/typescript-demo